LeetCode 131.分割回文串
分割回文串
给你一个字符串 s
,请你将 s
分割成一些子串,使每个子串都是 回文串 。返回 s
所有可能的分割方案。
回文串 是正着读和反着读都一样的字符串。
输入:s = "aab" |
方法:回溯
算法思路:
切割问题类似于组合问题,例如对于字符串abcdef:
- 组合问题:选取一个a之后,在bcdef中再去选取第二个,选取b之后在cdef中在选组第三个……。
- 切割问题:切割一个a之后,在bcdef中再去切割第二段,切割b之后在cdef中在切割第三段……。
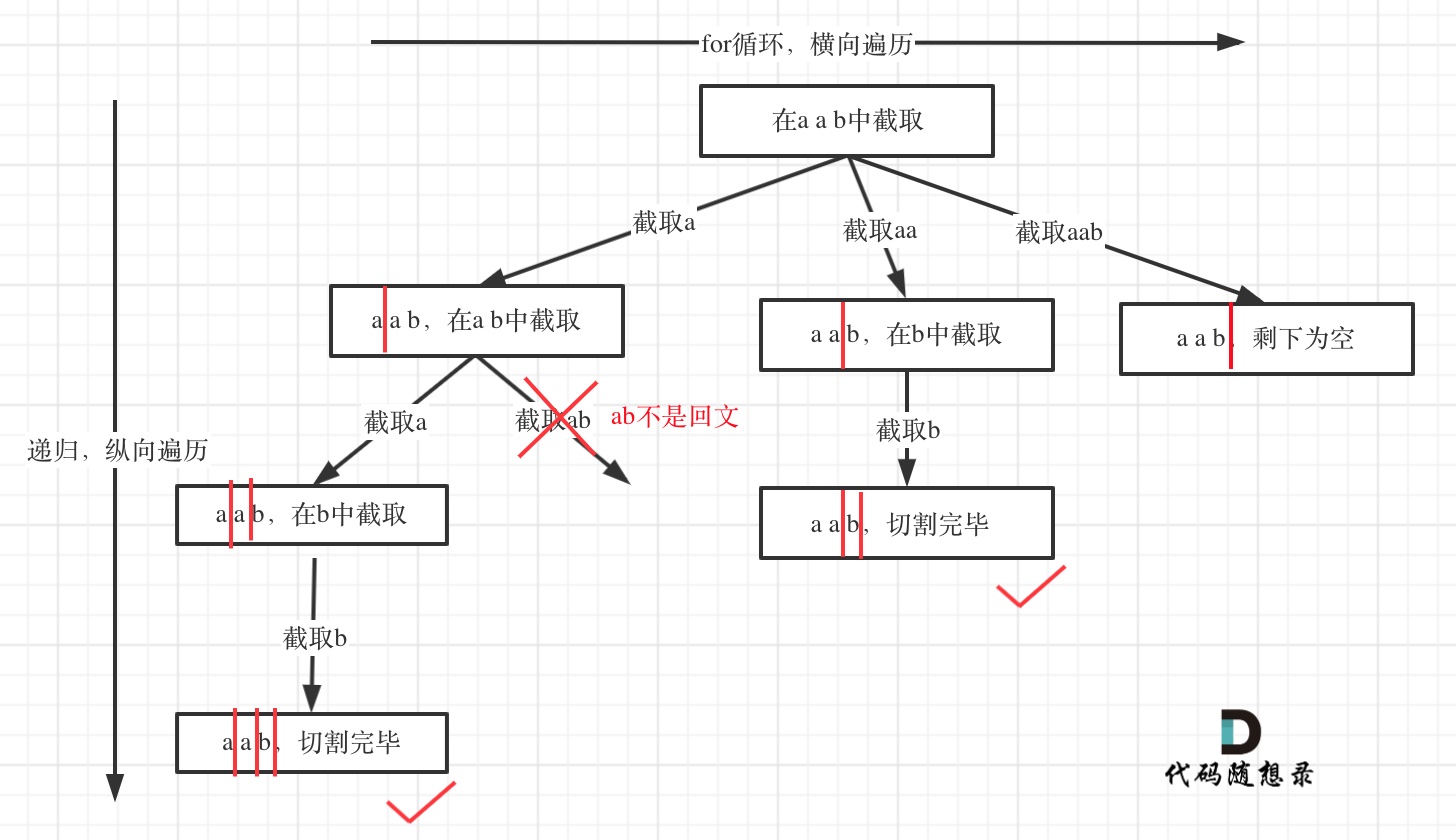
-
确定递归函数参数
除了输入的字符串
s
外,还需设置startIndex
记录切割过的位置,不能重复切割。 -
确定终止条件
当切割线到字符串最后面,即
startIndex == s.size()
时,终止递归。if(startIndex == s.length()){
result.add(new ArrayList<>(path));
return;
} -
编写判断是否为回文串的代码
public boolean isPalindrome(String s, int startIndex, int end){
while(startIndex < end){
if(s.charAt(startIndex++) != s.charAt(end--)){
return false;
}
}
return true;
} -
单层搜索的过程
判断
startIndex
到i
的子串是否为回文串,若是则加到path
中,否则continue
结束本次for
循环。
代码实现:
class Solution { |
参考
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来自 Abacteria的学习小站!