LeetCode 216.组合III
组合III
找出所有相加之和为n
的k
个数的组合,且满足下列条件:
-
只使用数字1到9
-
每个数字最多使用一次
返回所有可能的有效组合的列表 。该列表不能包含相同的组合两次,组合可以以任何顺序返回。
plaintext
输入: k = 3, n = 9 |
方法一:回溯
算法思路:
-
确定递归函数的返回值以及参数
除了
n
与k
,我们还需要设置变量sum
记录path
中元素的和,startIndex
记录循环搜索的起始位置 -
确定终止条件
当
path
大小达到k
时终止递归,若此时path
中的元素和为sum
等于n
,则在result
中添加path
javaif(path.size() == k){
if(sum == n){
result.add(new ArrayList<>(path));
}
return;
} -
单层搜索的过程
和77.组合一样,都是从
startIndex
开始遍历,其中sum
来统计path
中的元素之和,回溯后需要减回去。javafor(int i = startIndex; i <= 9; i++){
path.add(i);
sum += i;
backTracking(k, n, i + 1, sum);
sum -= i;
path.removeLast();
}
代码实现:
java
class Solution { |
方法二: 剪枝优化
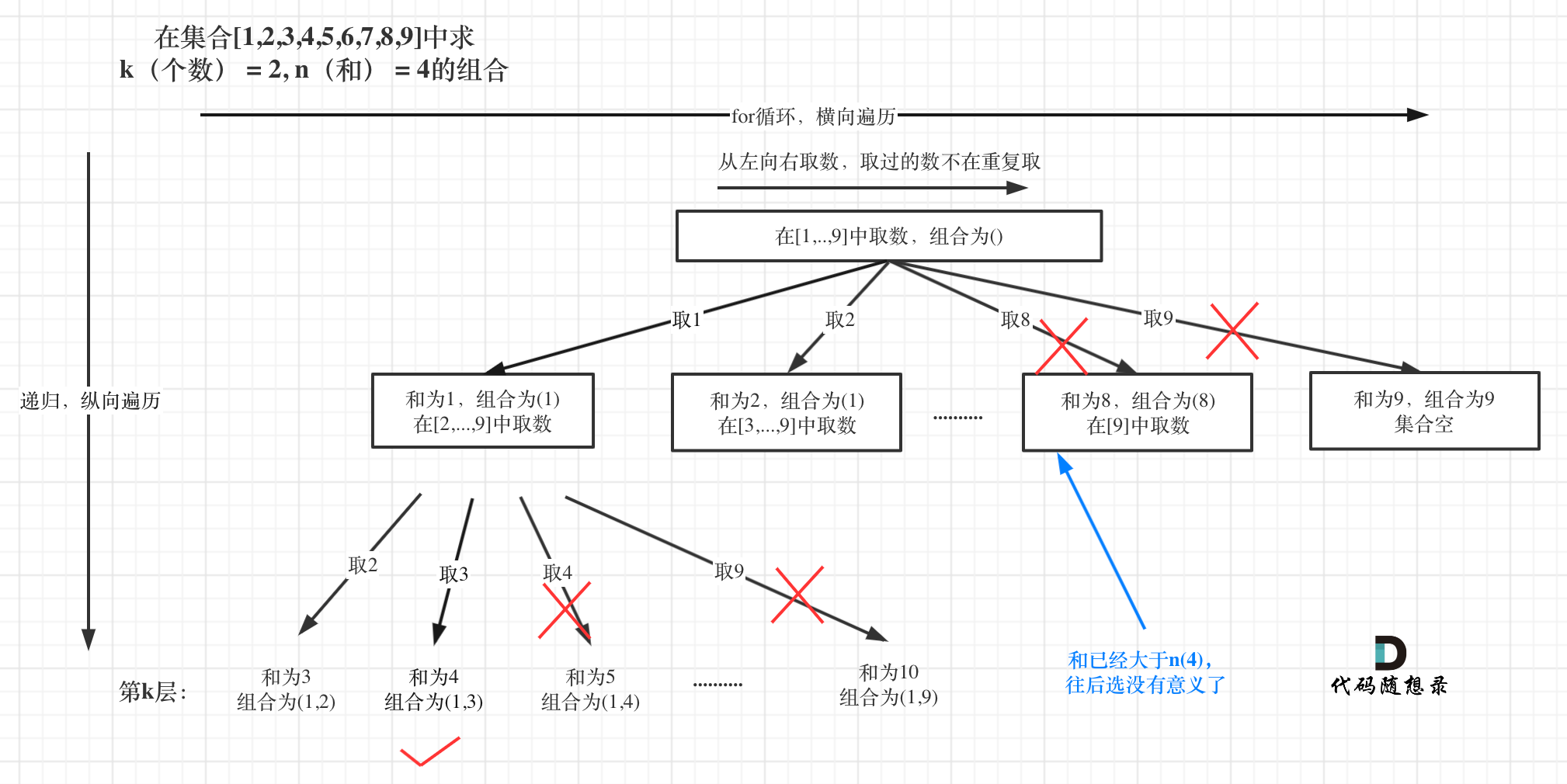
当元素总和已经大于n
时,往后遍历就没有意义了,直接剪掉。
java
if(sum > n){ |
与77.组合一样,for
循环也可以剪枝。
java
for(int i = startIndex; i <= 9 - (k - path.size()) + 1; i++) |
参考
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来自 Abacteria的学习小站!