LeetCode 206.反转链表
反转链表
给你单链表的头节点 head
,请你反转链表,并返回反转后的链表。
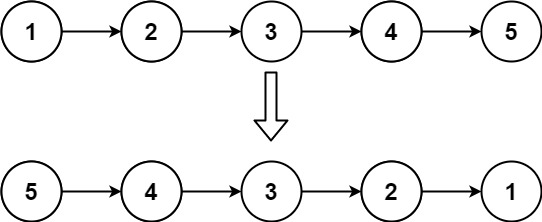
输入:head = [1,2,3,4,5] |
方法一
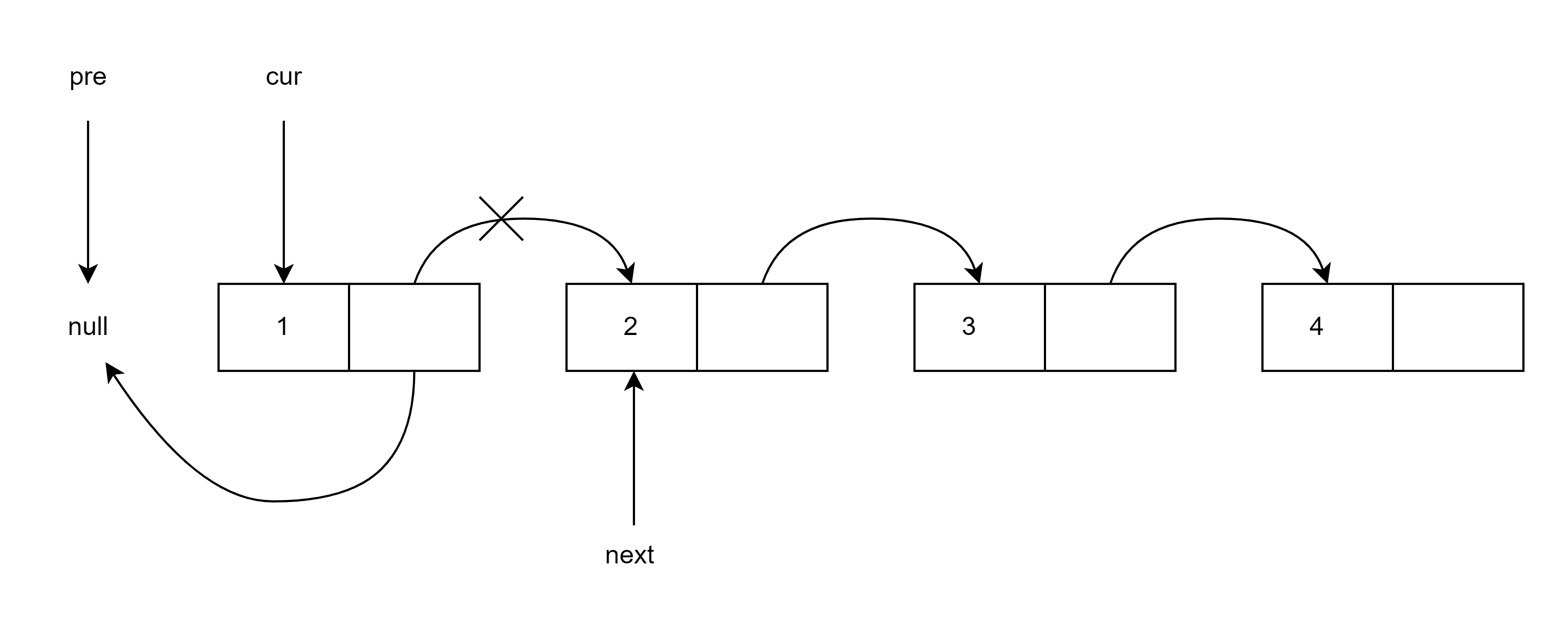
算法思路:
- 将
next = cur.next
储存起来 cur.next = pre
pre
与cur
向后移
代码实现:
class Solution { |
复杂度分析:
- 时间复杂度:,其中是链表的长度,需要遍历链表一次。
- 空间复杂度:。
方法二:递归
假设链表为:
1→2→3→4→5
某一时刻,我们处于如下状态:
1→2→3←4←5
我们希望3
的下一个节点指向2
,所以2.next.next = 2
。
需要注意的是,头结点的下一个节点必须指向,不然可能会产生环。
代码实现:
class Solution { |
复杂度分析:
-
时间复杂度:,其中 是链表的长度。需要对链表的每个节点进行反转操作。
-
空间复杂度:,其中 是链表的长度。空间复杂度主要取决于递归调用的栈空间,最多为 层。
参考
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来自 Abacteria的学习小站!